Online JavaScript Training Course for Beginners | GoSkills
Introduction to JavaScript
Skills you’ll gain
The JavaScript programming language is a fundamental building block of the internet, and is an essential tool to have in your web development toolkit. This online JavaScript training course can help you use JavaScript in conjunction with HTML and CSS to add interactive, dynamic elements to your web pages.
Designed for beginners, no prior experience or special skills are required to take this course. You will learn the basics of JavaScript for front end web development through hands-on application and examples every step of the way. By the end of the course, you will have built a fully functional flashcard app to demonstrate your practical knowledge.
Highlights:
- 40 practical tutorials.
- Learn the syntax and output of JavaScript code.
- Understand JavaScript statements and learn how to comment code.
- Store data in variables and apply arithmetic, comparison and logic operators.
- Understand the difference between strings, numbers, and Boolean data types.
- Utilize arrays to contain multiple items.
- Master intermediate JavaScript such as objects, functions and conditional statements.
- Make changes to HTML elements by manipulating the DOM.
- Make changes to CSS styles on a web page.
- Understand how to use Events and EventListeners.
- Add validation to an HTML fill-out form.
- Learn about JQuery, a popular JavaScript Library.
- Understand AJAX requests and responses.
- Put together everything you've learned by building a math flashcard app with JavaScript and AJAX.
Once enrolled, our friendly support team and tutors are here to help with any course related inquiries.
Syllabus
Download syllabus-
1
Statements and Comments Understanding the instructions that are executed by the web browser, and learning how to comment code. 4m
-
2
Variables How to store data in variables in JavaScript. 4m
-
3
Assignment Operators How to assign values to a variable, and do basic math assignment. 3m
-
4
Arithmetic Operators How to add, subtract, multiply, and divide with JavaScript. We'll also look at the modulus, incrementing and decrementing. 3m
-
5
Comparison and Logic Operators How to compare two or more variables and test with logic (and, or, not). 4m
-
6
Strings, Numbers, and Boolean Data Types Understanding the difference between strings and numbers; and understanding Boolean data types. 3m
-
7
Arrays What are arrays, and how does JavaScript use them? 4m
-
1
Objects What are objects, and how does JavaScript use them? 3m
-
2
Functions Implementing and invoking blocks of code with functions. 3m
-
3
If and If/Else and If/Else If Statements Understanding conditional statements using If, If Else, and If Else If statements. 4m
-
4
Switch Understanding conditional statements using switch. 3m
-
5
For Loops Looping through things using For loops. 3m
-
6
While Loops Looping through things using While loops. 4m
-
7
Intro to JSON Introduction to JSON and why it is important. 4m
-
1
A Short HTML/CSS Primer JavaScript works with HTML and CSS, let's do a quick refresher video on HTML and CSS. 3m
-
2
Intro to the HTML DOM What is the DOM and why is it important? 3m
-
3
DOM Methods and Properties What are the actions you can perform on HTML elements and what properties can you get and set? 3m
-
4
Dom Document Objects Finding, changing, adding, and deleting elements. 3m
-
5
Finding Dom Elements Finding elements by ID, by tag name, by class name, by CSS selectors, and by collections. 4m
-
6
Changing HTML Output, Content, and Attributes How to change HTML output, content, and attributes with JavaScript. 3m
-
7
Changing CSS How to change your CSS with JavaScript. 4m
-
8
Understanding Events What is an event, and what can you do with it? 4m
-
9
Event Listeners What are event listeners, and how to listen for event handlers. 4m
-
10
Navigating DOM Nodes What is a DOM node and how do you navigate them. 4m
-
11
Creating New Nodes Adding new nodes on the fly with JavaScript. 3m
-
12
The Node List How to retrieve an array-like collection of nodes. 4m
-
13
Form Validating How to validate an HTML fill-out form with JavaScript. 3m
-
14
Introduction to jQuery What is jQuery, and why is it important? 4m
-
1
What Is AJAX What is AJAX and what is it used for? 3m
-
2
AJAX Requests - GET or POST? What's the difference between GET and POST and when should you use them? 3m
-
3
AJAX Response How to handle an AJAX response. 3m
-
1
Putting It All Together - What We'll Build Introduction to building a simple math flashcard app with JavaScript and Ajax. 3m
-
2
Create the Fill-Out Form Let's create a fill-out form to enter our answers. 4m
-
3
Checking for Numbers Let's make sure the user entered a number! 3m
-
4
Output the Result How to output the answers to the screen. 4m
-
5
Finishing Up Create pages for Subtraction, Multiplication, and Division and finish the app. 5m
Certificate
Certificate of Completion
Awarded upon successful completion of the course.

Instructor
John Elder
John founded one of the Internet's earliest advertising networks (bannerclicks.com) and sold it at the height of the first dot com boom. John went on to develop the award-winning Submission-Spider search engine submission software that's been used by over 3 million individuals, businesses, and governments in over 42 countries.
John has over 20 years experience in web development, building professional websites across all platforms. John's passion for learning new technologies lead him to master both front end and back end work, making him a sought after full-stack developer.
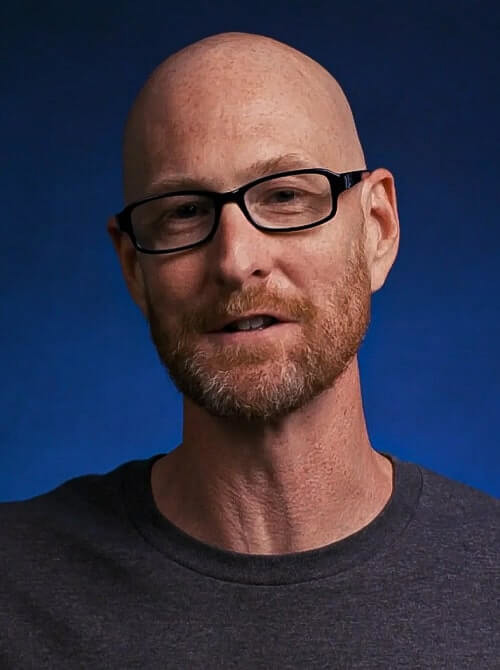
John Elder
Web Developer and Author
Accreditations
Link to awardsHow GoSkills helped Chris
I got the promotion largely because of the skills I could develop, thanks to the GoSkills courses I took. I set aside at least 30 minutes daily to invest in myself and my professional growth. Seeing how much this has helped me become a more efficient employee is a big motivation.
