Best Excel Macros & VBA Online Training Courses - {Date:mmmm} {Date:yyyy} Update
Microsoft Excel - Macros and VBA
Skills you’ll gain
Learn to automate your most repetitive tasks at the push of a button with Excel macros and VBA. In this online course, you will learn to create powerful macros using VBA (Visual Basic for Applications) to really make Excel work for you. With no prior programming experience required, you'll have your daily spreadsheets running like clockwork to save you hours of time and boost your productivity.
Highlights:
- 41 practical tutorials.
- Understand the differences between macros and VBA.
- Run your macro from the Macro dialog, a keyboard shortcut or worksheet button.
- Understand the syntax and structure that make up a valid macro.
- How VBA Objects and the Objects model relate to each other.
- Using step vs run in entry level debugging.
- How to create variables and assign strings, values or objects.
- Implementing logic tests in VBA.
- Set up looping with Do loops and Do While loops.
- Create a VBA Message Boxes.
- Set up an error trap in VBA to handle errors.
- Create a User Defined Function (UDF).
Video tutorials are recorded in Microsoft Excel 2016 for PC. Learn more about how a GoSkills Excel certification can boost your career.
Want to be a more efficient Excel user? Start learning 200 of the best Excel shortcuts for PC and Mac.
Once enrolled, our friendly support team and tutors are here to help with any course related inquiries.
Syllabus
Download syllabus-
1
Creating Your First Macro Recording a simple Macro in Excel. 4m
-
2
File Types & Saving A discussion on which files types support macros, and why it is critical to save your work before moving forward. 4m
-
3
Running Macros: Using the Macro Dialog How to run a macro from the Macro dialog. 5m
-
4
Running Macros: Using Keyboard Shortcuts How to run a macro from a keyboard shortcut. 5m
-
5
Running Macros: Using Buttons How to run your macro from a worksheet button. 5m
-
1
Navigating the Visual Basic Editor Meet the Visual Basic Editor (VBE) - your coding studio. 5m
-
2
VBA Objects & the Object Model A discussion of the different items you'll encounter as you learn to code, and how they relate to each other. 5m
-
3
Excel's VBA Object Model A visual view of a portion of Excel's object model. 5m
-
4
Where Do I Put My Code? Examining the different code containers and where you should place your code. 4m
-
5
Understanding Code: Macro Syntax Looking at the different keywords and structure that make up a valid macro. 4m
-
6
Cleaning up Recorded Code Editing the previously recorded code in order to remove unnecessary objects. 6m
-
1
Step vs Run How to step through a macro line by line in order to aid in debugging. 5m
-
2
Using Breakpoints How to set and use breakpoints during code development and debugging. 5m
-
1
What are Variables? A discussion of what variables are, and what they do for us when coding. 5m
-
2
Creating Variables Setting up variable dimensions and ensuring the code does so in the correct location. 5m
-
3
Setting Variables How to assign strings, values or objects to variables and use them in your code. 7m
-
4
Explicit vs Implicit Variables Why forgetting to set a simple flag can burn you in the long term. 6m
-
1
Using the Locals Window Working with the locals window to help you debug and explore the object model. 5m
-
2
Using the Immediate Window Exploring the benefits of the Immediate window for logging and querying, as well as writing when needed. 6m
-
3
Using the Watch Window How to use the Watch window to break code execution when variable conditions are met. 5m
-
4
Using the Stop Keyword How the Stop keyword can be used during code development similar to a breakpoint. 5m
-
1
With Blocks How "With" blocks can tighten up your code and ensure your code targets the objects you expect. 5m
-
2
Logic Tests: If Then Else Implementing If/Then choices in VBA. 6m
-
3
Logic Tests: Select Case Understanding how the Select Case construct adds another logic test to your coding arsenal. 6m
-
4
Loops: Basic Looping with Do Loops Basic looping including counting iterations and exiting. 5m
-
5
Loops: Looping under Conditions with Do While/Until Loops More advanced looping by looping while or until a certain condition is met. 5m
-
6
Loops: Looping X Iterations with For X to Y Loops Running a loop a set number of times. 5m
-
7
Loops: Looping Through Collections with For Each X in Y Loops Using a For Each loop to cycle through each object in a collection such as each worksheet in a workbook's worksheets. 5m
-
8
Calling Other Macros Setting up a master macro allowing you to call other macros from a single source. 6m
-
1
Creating VBA Message Boxes How to provide feedback to your user via the VBA MsgBox object. 5m
-
2
Collecting Feedback from a VBA MsgBox Identifying which button the user clicked when presented with a MsgBox in order to use their response in our code. 6m
-
3
Collecting Feedback from a VBA InputBox Working with the VBA InputBox to prompt the user to enter information and capture it for later use. 5m
-
4
Forcing User Input Forcing users to enter data when requested. 5m
-
1
Error Types How to trigger various errors in VBA, and what they mean. 5m
-
2
Trapping and Handling Errors How to set up an error trap in VBA to handle errors. 5m
-
3
Building Error Handlers Setting up an error handling section for your macro. 5m
-
1
User Defined Function (UDF) Syntax The syntax signature for a UDF and how it differs from a standard subroutine. 3m
-
2
Creating User Defined Functions (UDFs) Creating a UDF to return the user name. 5m
-
3
Calling a User Defined Function (UDF) Calling a UDF from a worksheet and from VBA. 5m
Certificate
Certificate of Completion
Awarded upon successful completion of the course.

Instructor
Ken Puls
Ken Puls is a globally recognized authority in Microsoft Excel, Power Query, and Power BI, with over two decades of hands-on experience in accounting, IT, and business intelligence systems. His career began in public practice accounting and industry, where he served as both Controller and IT Director, leading numerous system implementations and designing custom business intelligence solutions from the ground up.
Ken’s deep technical expertise is matched by a passion for teaching and community engagement. He has authored several acclaimed books, including RibbonX – Customizing the Office 2007 Ribbon, Power Query Recipes, and Master Your Data with Power Query in Excel and Power BI. His writing reflects a clear, practical approach to solving real-world data challenges.
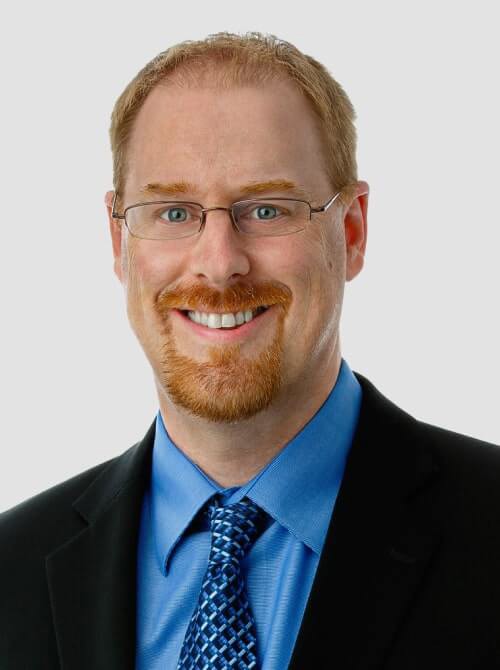
Ken Puls
Excel MVP
Accreditations
Link to awardsHow GoSkills helped Chris
I got the promotion largely because of the skills I could develop, thanks to the GoSkills courses I took. I set aside at least 30 minutes daily to invest in myself and my professional growth. Seeing how much this has helped me become a more efficient employee is a big motivation.
