Intro to Python Course Online | Training for Beginners - GoSkills
Introduction to Python
Skills you’ll gain
Python is one of the world's most popular, fastest-growing programming languages. It's versatile enough to build apps for data analysis all the way to building robots with Raspberry Pi.
You don't need any previous experience writing code to take this Intro to Python course online. When you’re finished you will understand the fundamentals of the object-oriented Python language, and you’ll be able to write your own basic programs.
Why learn Python?
This Intro to Python training for beginners will teach you the fundamentals, so that you'll be ready to jump into the world of programming with confidence.
In this Python course online you will learn how to:
- Install Python on your operating system
- Understand data types including strings, numbers, lists, tuples, variables, and dictionaries
- Use assignment operators
- Convert data types
- Use comparison operators and conditional statements like if, else, and elif
- Use membership and identity operators
- Import and create your own modules
- Write loops and loop control statements like while and for loops
- Write functions
- Open, close, rename and delete files
- Create and initialize classes, and calling attributes
- Use class inheritance for classes to interact with each other
Once enrolled, our friendly support team and tutors are here to help with any course related inquiries.
Syllabus
Download syllabus-
1
Data Type Conversion How to convert one data type into another data type. 4m
-
2
Comparison Operators How to compare two things. 3m
-
3
Conditionals If/Else/Elif Using comparison operators to make decisions. 4m
-
4
Multiple Conditionals What are the logical operators AND/OR/NOT and how do we use them? 3m
-
5
Python Formatting Formatting in Python is important! Let's look at lines and indentation, and single line If statements. 4m
-
6
Membership Operators We'll look at the membership operators "In", and "Not In". 3m
-
7
Identity Operators We'll look at the identity operators "Is", and "Is Not". 3m
-
8
While Loops Learn to loop using a While loop. 4m
-
9
For Loops Learn to loop using a For loop. 3m
-
10
Loop Control Statements Take control of your loops using "break", "continue", and "pass". 4m
-
11
FizzBuzz! Let's build a FizzBuzz app! 4m
-
12
Functions Part 1 What are functions and how to use them. 4m
-
13
Functions Part 2 More about functions and how to use them. 4m
-
14
Program Flow Understanding Program Flow in Python, and how functions can change that flow. 4m
-
15
Random Numbers How to create and use random numbers. 4m
-
16
Modules Using third party modules in your code, and creating your own. 4m
-
17
Getting User Input Allow the user to interact with your program with raw_input and input. 3m
-
18
Opening and Closing Files How to open and close a file with Python. 5m
-
19
Reading and Writing Files How to read and write to a file with Python. 3m
-
20
Renaming and Deleting Files How to rename and delete a file with Python. 3m
-
1
Overview of Classes and Object Oriented Programming What are classes and what are they used for. 4m
-
2
Creating a Class Part 1 How to create a simple class. 4m
-
3
Creating a Class Part 2 More about creating simple classes. 4m
-
4
Creating A Class Part 3 Finishing up our tutorial on classes. 4m
-
5
Built-In Class Attributes What are the built-in Python class attributes? 4m
-
6
Class Inheritance Using other classes inside of your class. 4m
-
1
Install Sublime and Git Bash Terminal We'll start off by installing the Sublime Text editor and the Git Bash Terminal. 4m
-
2
First Program Let's create our first program. 4m
-
3
Comments and C9 Transition Learn when and how to use comments in your code. 6m
-
4
Installing Python We'll download and install Python. 3m
Certificate
Certificate of Completion
Awarded upon successful completion of the course.

Instructor
John Elder
John founded one of the Internet's earliest advertising networks (bannerclicks.com) and sold it at the height of the first dot com boom. John went on to develop the award-winning Submission-Spider search engine submission software that's been used by over 3 million individuals, businesses, and governments in over 42 countries.
John has over 20 years experience in web development, building professional websites across all platforms. John's passion for learning new technologies lead him to master both front end and back end work, making him a sought after full-stack developer.
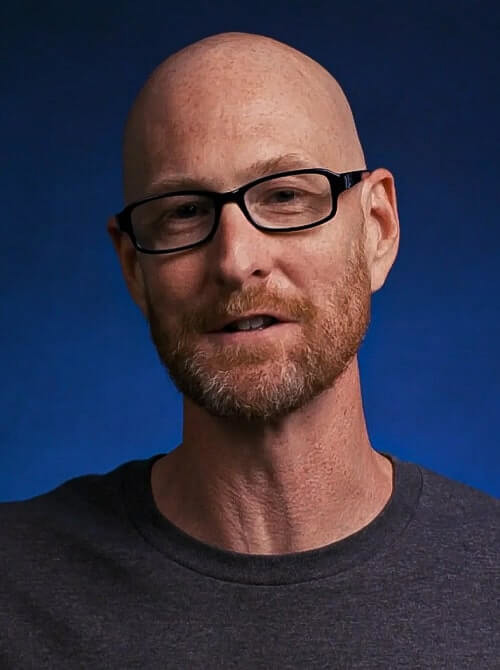
John Elder
Web Developer and Author
Accreditations
Link to awardsHow GoSkills helped Chris
I got the promotion largely because of the skills I could develop, thanks to the GoSkills courses I took. I set aside at least 30 minutes daily to invest in myself and my professional growth. Seeing how much this has helped me become a more efficient employee is a big motivation.
