Intro to Ruby Programming
Intro to Ruby Programming
Skills you’ll gain
If you have ever wanted to learn Ruby online, this intro to Ruby programming course can help you get started building your own web apps. Ruby is known for being a fun and comparatively simple language to learn, ideal for beginners and aspiring web developers. Proficiency in Ruby and its accompanying framework, Ruby on Rails, is a marketable skill, with growing demand for Ruby and Rails developers in the job market.
Designed for beginners, this intro to Ruby course starts out helping you learn the basics of Ruby programming, including operators, variables and conditional statements. You will build on this foundation with intermediate and advanced concepts in Ruby, with practical, hands-on application and examples every step of the way. By the end of the course, you will apply your skills to build a math flashcard game that you can add to your portfolio, and gain the fundamental knowledge you need to start creating more projects with Ruby.
Highlights:
- 41 practical tutorials.
- Store data in variables and apply arithmetic, comparison and assignment operators.
- Learn how to comment code and deal with errors.
- Create and use arrays and multi-dimensional arrays.
- Understand how and when to use loops, including while, until, for and each loops.
- How to create, use and manipulate hashes.
- Utilize classes, class getters and class setters.
- Learn different modes for opening files and how to write and append data to a file.
- Install and use a third party Gem in your program for extra functionality.
- Put together everything you've learned by building a math flashcard app.
Want to continue improving your skills in Ruby? Check out the Ruby on Rails course.
Once enrolled, our friendly support team and tutors are here to help with any course related inquiries.
Syllabus
Download syllabus-
1
Comments and Errors How to comment code and deal with errors. 4m
-
2
Arithmetic Operators How to do basic math with Ruby. 3m
-
3
Floats and Integers Understanding the difference between floats and integers and when to use each. 3m
-
4
Comparison Operators How to compare two or more items with Ruby. 3m
-
5
Variables Storing information in variables is easy! 4m
-
6
Assignment Operators How to assign items to variables (and other things). 3m
-
7
Getting User Input with Gets Allowing the user to interact with your program. 3m
-
8
Conditional Statements How to use if/else/elsif statements in Ruby. 4m
-
9
Multiple Conditional Comparison Operators Sometimes you need to compare more than two things, here's how. 3m
-
10
String Manipulation Modifying a string is simple with these string manipulation tips. 3m
-
1
Arrays What are arrays and how do we create and use them? 3m
-
2
Multi-Dimensional Arrays An array of other arrays is multi-dimensional. 3m
-
3
While Loops Using while loops in Ruby. 3m
-
4
Until Loops Looping until a condition is met using until loops. 4m
-
5
For and Each Loops Understanding for and each loops, and when to use them. 3m
-
6
FizzBuzz! Fizzbuzz! is a popular interview question/quiz. Let's build it! 4m
-
7
Hashes What is a hash, and how do you create and use it? 3m
-
8
Hash Manipulation Adding and removing items from a hash. 3m
-
9
Methods Understanding methods in Ruby. 3m
-
10
Methods Part 2 More on methods... 5m
-
11
Random Numbers How to generate random numbers with Ruby. 3m
-
1
Classes What is a class and how do we use them? 4m
-
2
Classes Part 2 More on classes and how to use them. 3m
-
3
Instance Variables What is an instance variable and how is it different from a regular variable? 3m
-
4
Class Getters What is a getter and how do we use it? 3m
-
5
Class Setters How to create a class setter and why you should. 3m
-
6
Understanding Attr_accessor Let Ruby create your getters and setters automatically with attr_accessor. 3m
-
7
Class Inheritance Inheriting information from other classes. 3m
-
8
Opening a File How to open another file in your Ruby file. 4m
-
9
Open File Modes There are several "modes" for opening files. Learn them all here. 3m
-
10
Adding Third-party Functionality with Gems How to install and use a third-party gem in your program. 4m
-
11
Open a File into an Array We'll learn to output the contents of a file into an array. 3m
-
12
Write to a File We'll learn to write and append data to a file. 3m
-
1
Building a Flashcard Game Let's use what we've learned to build a math flashcard game! 3m
-
2
Flashcard Addition Method How to handle the addition flashcards. 4m
-
3
Flashcard Subtraction, Multiplication, Division Methods How to handle subtraction, multiplication and division flashcards. 4m
-
4
Creating a Menu Allow the user to choose which type of flashcard game to play with a menu. 5m
Certificate
Certificate of Completion
Awarded upon successful completion of the course.

Instructor
John Elder
John founded one of the Internet's earliest advertising networks (bannerclicks.com) and sold it at the height of the first dot com boom. John went on to develop the award-winning Submission-Spider search engine submission software that's been used by over 3 million individuals, businesses, and governments in over 42 countries.
John has over 20 years experience in web development, building professional websites across all platforms. John's passion for learning new technologies lead him to master both front end and back end work, making him a sought after full-stack developer.
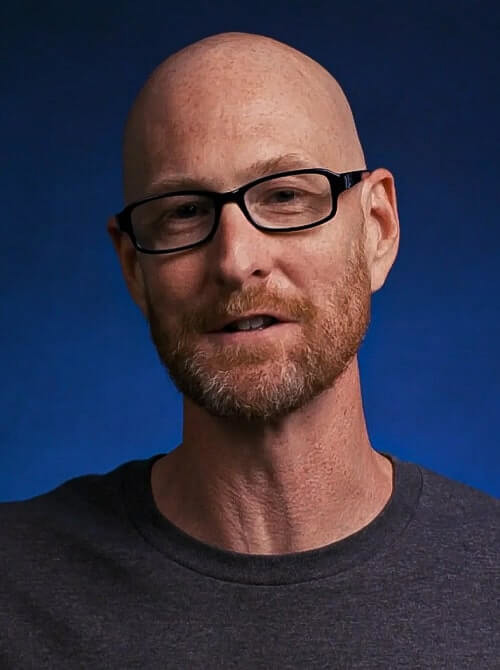
John Elder
Web Developer and Author
Accreditations
Link to awardsHow GoSkills helped Chris
I got the promotion largely because of the skills I could develop, thanks to the GoSkills courses I took. I set aside at least 30 minutes daily to invest in myself and my professional growth. Seeing how much this has helped me become a more efficient employee is a big motivation.
