Learn C# | C# course for beginners
Intro To C# Programming
Skills you’ll gain
C# is a powerful cross-platform, object-oriented programming language used by many programmers to create apps for mobile and desktop.
Designed for beginners, this intro to C# course teaches you the basics of C# programming, including variables, data types, and math operations. You will build on this foundation with practical, hands-on exercises and examples every step of the way. You will be able to apply your skills to create programs of your own - like a fun Madlib game - that you can add to your portfolio. By the end of the course, you will have gained the fundamental knowledge you need to start creating more projects with C#.
In this intro to C# course, you will learn how to:
- Explore fundamental concepts including variables, data types, strings, and math operations
- Collect user input
- Work with arrays, methods, logic, and loops
- Ensure proper error and exception handling
- Get an introduction to classes
- Build your own basic program and more!
Once enrolled, our friendly support team and tutors are here to help with any course-related inquiries.
Syllabus
Download syllabus-
1
Variables An introduction to the concept of Variables and how we will use them in C# 5m
-
2
Data Types: Strings, Char, Int, float, double, decimal, boolean We introduce many of the Data Types you can use: Strings, Char, Int, float, double, decimal, and boolean. 7m
-
3
Datetime We introduce the Datetime data type and explain how to set it to a specific value. 2m
-
4
Null / Nullables We explain the concept of Null, discuss which fields can have a null value, and introduce Nullables. 3m
-
5
String Methods We examine various String Methods to modify string variables, including changing the case and replacing parts of the string. 4m
-
6
String Indexing We explain the concept of String Indexing to manage and modify elements within a string. 3m
-
7
String Concatenation and Interpolation We learn the difference between Concatenation and Interpolation, and when one might be easier to use than the other. 3m
-
8
Math (Addition, Subtraction, Multiplication, Division) Let's learn how to perform basic math functions: Addition, Subtraction, Multiplication, Division. 4m
-
9
Math (Exponents and Modulus) In this lesson, we examine how Exponents work and what the Modulus is. 3m
-
10
Math Order Of Operations We review PEMDAS and how the Order of Operations works within C#. 5m
-
11
Math Floats Vs. Ints In this lesson, we discuss the differences between integers, floats, decimals, and doubles, and how decimal places may be affected based on the data type you choose. 3m
-
12
Math Incrementation ++ and -- We learn how to increment and decrement variables using ++ and -- commands. 4m
-
13
Math Methods In this lesson, we learn about Math Methods such as Floor, Ceiling, Round, and Truncate. 5m
-
14
DateTime Functions Let's explore the various functions we can use to display and use the DateTime data type. 4m
-
1
Converting Integers To Strings In this lesson, we discuss how to convert Integers to Strings using the toString method. 3m
-
2
Type Conversion/Casting We examine the process for Type Conversion and Casting. 4m
-
3
User Input / Output In this lesson, we learn how to capture User Input, assign it to a variable, and Output it to the screen. 3m
-
4
Build a Madlib Program! Let's practice our skills with user input to build a Madlib Program! 3m
-
5
Creating and Accessing Arrays In this lesson, we learn how to create and access Arrays. 6m
-
6
Updating Arrays We explore how to update an existing Array by changing one of its values. 3m
-
7
Appending Arrays Appending Arrays in C# is a bit different than in other programming languages. 3m
-
8
Two Dimensional Arrays In this lesson, we examine how to create and use Two Dimensional Arrays. 4m
-
9
Methods In this lesson, we introduce Methods and how to use them in your program. 5m
-
10
Passing Parameters To Methods Once you've created a method, you may want to pass Parameters to those Methods to perform specific operations. 4m
-
11
Return Methods If you'd like to use Methods and return data to your program, we explore how that's done. 4m
-
12
Logic: Comparison Operators (>, >=, <, <=, !=, ==) In this lesson, we learn about Logic and basic Comparison Operators: >, >=, <, <=, !=, ==. 5m
-
13
Logic: If/Else Statements We introduce If/Else Statements and look at how they run inside a program. 3m
-
14
Logic: If/Else And Operators In this lesson, we learn how to use "And" operators within an If/Else statement. 3m
-
15
Logic: If/Else Or Operators We learn how to use "or" operators within If/Else statements. 3m
-
16
Logic: If/Else If In this lesson, we learn how to use "else if" within an If/Else statement. 3m
-
17
Switch Statement We look at how to use a Switch Statement within our program. 4m
-
18
Loops: While Loops In this lesson, we introduce Loops and explain how While and Do While work. 4m
-
19
Loops: For Loops We learn how to create and use For Loops within our program. 4m
-
20
Loops: Break and Continue In this lesson, we explain how to Break and Continue loops. 4m
-
1
Error and Exception Handling We discuss how to deal with Errors within your code and how Exception Handling can prevent your program from crashing. 4m
-
2
Catching Specific Exceptions By anticipating specific kinds of Exceptions, you can program your code to treat them in unique ways without crashing. 6m
-
3
Finally Exception Handling The Finally part of Exception Handling will ensure specific code runs after try / catch blocks. 3m
-
4
Exception Lists In this lesson, we identify a helpful Exception List that can help educate you on potential issues that could impact your code. 4m
-
1
Intro To Classes (Part 1) Let's begin a discussion of object-oriented programming so we can create our own data types. 3m
-
2
Intro To Classes (Part 2) We learn how to create our own class and define the attributes within. 6m
-
3
Intro To Classes: Constructors Constructors provide a simple way to create new objects within our code. 6m
-
4
Intro To Classes: Object Methods In this lesson, we learn how to create Object Methods that operate inside your own classes. 5m
Certificate
Certificate of Completion
Awarded upon successful completion of the course.

Instructor
John Elder
John founded one of the Internet's earliest advertising networks (bannerclicks.com) and sold it at the height of the first dot com boom. John went on to develop the award-winning Submission-Spider search engine submission software that's been used by over 3 million individuals, businesses, and governments in over 42 countries.
John has over 20 years experience in web development, building professional websites across all platforms. John's passion for learning new technologies lead him to master both front end and back end work, making him a sought after full-stack developer.
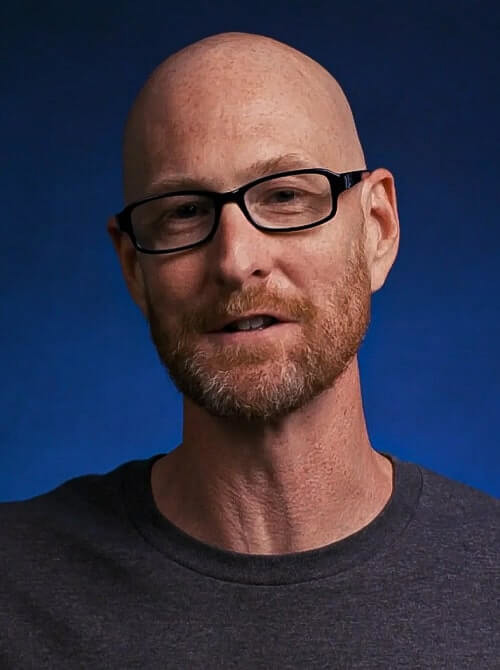
John Elder
Web Developer and Author
Accreditations
Link to awardsHow GoSkills helped Chris
I got the promotion largely because of the skills I could develop, thanks to the GoSkills courses I took. I set aside at least 30 minutes daily to invest in myself and my professional growth. Seeing how much this has helped me become a more efficient employee is a big motivation.
