Best Python Excel Course - Python with Excel Tutorial | GoSkills
Python with Excel
Skills you’ll gain
In this beginner-friendly course, you will learn how to use Python with Excel in tandem to drastically improve your data crunching and analysis skills.
In 40 short lessons, you will combine the power of Python and Excel using openpxyl to create, manipulate, and present data.
By the end of the course, you will be able to create spreadsheets, crunch data, and present information as charts and graphs solely using Python commands.
Using Python for Excel is ideal for those who work closely with databases or large spreadsheets, or who are interested in learning more about data analytics.
The course only requires a basic understanding of Excel and doesn’t require any previous Python or coding experience.
In this course, you will learn how to:
- Download and install Python, Sublime Text, Git Bash, and Python openpxyl
- Create your first python program
- Use variables, print (), lists, tuples, and loops
- Create workbooks and worksheets using Python
- Read and open Excel files in Python
- Use Excel formulas in the Python terminal
- Manipulate data and spreadsheets using Python
- Format and style spreadsheets using Python
- Create basic and advanced charts and graphs
- Create and manipulate tables
- Add images to Excel spreadsheets using Python
Want to learn more Python skills? Check out the Intro to Python and Python for Data Analysis courses.
Syllabus
Download syllabus-
1
Pip Install openpyxl and import openpyxl is the Python library that we'll use to connect our Python code to Excel. 5m
-
2
Creating Workbooks and Worksheets To work with workbooks and worksheets in excel, we need to import some things from openpyxl. 5m
-
3
Load Existing Excel File and Grab One Cell To load data from an existing Excel spreadsheet, we need to import load_workbook from openpyxl. 5m
-
4
Existing Excel - Cell Range In this lesson, we'll discuss how to grab a whole range of cells. 5m
-
5
Existing Excel - Row Range Use this method when you want to grab an entire row - or a range of rows - from an Excel spreadsheet. 4m
-
6
Existing Excel - Iterate Thru Rows Let's discuss another method to iterate through rows in an Excel spreadsheet when you need a little more control. 3m
-
7
Existing Excel - Iterate Thru Columns Just like iterating through rows, we can also iterate through columns. 3m
-
8
Change Existing Cells - Method 2 There's more than one way to change data in a spreadsheet cell. This method is handy to use when looping using For Loops. 2m
-
9
Existing Excel - Column and Column Range We can grab an entire column of data from an Excel spreadsheet. 4m
-
10
Using Excel Formulas Using Excel formulas in our Python code is easy! 3m
-
1
Create Excel Spreadsheet Using Python and Random Numbers Let's take what we've learned so far and put it to use with an exercise! 6m
-
1
Merge Cells and Unmerge Cells In this lesson, we'll cover how to merge and unmerge cells. 4m
-
2
Cell Font Properties We can change the font color and sizing by using the font property. 5m
-
3
Cell Alignment In this lesson, we'll discuss methods to change the alignment of text in a cell. 5m
-
4
Cell Fill (Pattern Fill) Pattern Fill allows you to change the color and pattern of a cell. 4m
-
5
Cell Fill (Gradient Fill) We can easily change the gradient color of a cell in a spreadsheet. 3m
-
6
Borders and Sides In this lesson, we'll discuss how to change the border style and thickness of a spreadsheet cell. 5m
-
1
Intro To Charts And Graphs - Pie Charts In this lesson, we'll learn how to add Pie Charts to a spreadsheet. 6m
-
2
Intro To Charts and Graphs - Bar Charts, Line Charts, Area Charts, ScatterCharts There are many other basic charts you can use with openpyxl, such as bar charts, line charts, area charts, and scatter charts. 5m
-
3
Bar Charts - Vertical, Horizontal, Stacked, and 3D In this lesson, we'll look at Bar Charts - Vertical, Horizontal, Stacked, and 3D - in a little more detail. 5m
-
4
Area Charts - 2D and 3D In this lesson, we'll discuss how to add 2D and 3D Area Charts to your spreadsheet. 4m
-
5
Radar Charts Let's show how to add Radar Charts to your spreadsheet. 4m
-
6
Doughnut Charts In this lesson, we'll look at how to add Doughnut Charts. 5m
-
7
Surface Charts We'll discuss how to add Surface Charts to your spreadsheet. 3m
-
8
Bubble Chart Use these steps to add a Bubble Chart to your spreadsheet. 5m
-
9
Projected Pie Charts In this lesson, we'll look at Projected Pie charts, which are pie charts with some chunks pulled out and projected to the side. 3m
-
1
Tables - Part 1 Tables are good for sorting data manually in your spreadsheet, and for drilling down and re-imagining your data. 2m
-
2
Tables - Part 2 In this lesson, we'll build a Table. 5m
-
3
Adding Images We'll cover how to add images to your spreadsheet. 4m
-
1
Introduction We'll spend a few minutes discussing what we'll learn throughout the course. 4m
-
2
Download and Install Sublime Text We're going to use the Sublime Text Editor to write our code in this course. So in this lesson, we'll download and install Sublime Text. 3m
-
3
Download and Install Git Bash To run the Python programs that we write, we need a terminal. We'll be using the git bash terminal in this course, so let's show how to download and install Git Bash. 3m
-
4
Virtual Environment To use Python properly, we create a virtual environment. In this lesson, we'll explain how to set one up. 5m
-
5
Download and Install Python In this lesson, we'll download and install the Python programming language. 3m
Certificate
Certificate of Completion
Awarded upon successful completion of the course.

Instructor
John Elder
John founded one of the Internet's earliest advertising networks (bannerclicks.com) and sold it at the height of the first dot com boom. John went on to develop the award-winning Submission-Spider search engine submission software that's been used by over 3 million individuals, businesses, and governments in over 42 countries.
John has over 20 years experience in web development, building professional websites across all platforms. John's passion for learning new technologies lead him to master both front end and back end work, making him a sought after full-stack developer.
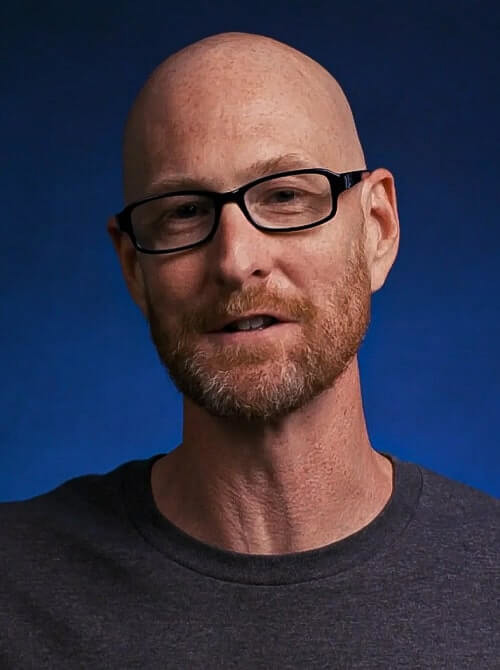
John Elder
Web Developer and Author
Accreditations
Link to awardsHow GoSkills helped Chris
I got the promotion largely because of the skills I could develop, thanks to the GoSkills courses I took. I set aside at least 30 minutes daily to invest in myself and my professional growth. Seeing how much this has helped me become a more efficient employee is a big motivation.
